- Geek's Newsletter
- Posts
- Revolutionize Your Code: Learn Python List Comprehensions Now!
Revolutionize Your Code: Learn Python List Comprehensions Now!
Mastering Python List Comprehensions: Elevate Your Code to Pythonic Excellence
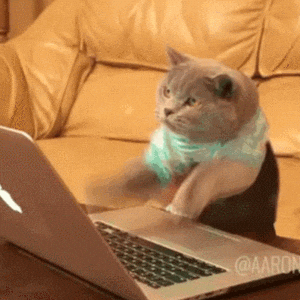
Mastering Python List Comprehensions: Code Your Way to Efficiency
Unleash the Power of Pythonic List Comprehensions
Dear Python Enthusiast,
Welcome to an immersive journey into the world of Python list comprehensions, where we'll unravel the art of crafting efficient, concise, and elegant code. By the time you reach the end of this newsletter, you'll be equipped with a formidable coding technique that can transform the way you work with Python.
Embracing List Comprehensions: A Game-Changer in Python
List comprehensions are more than just a Python feature; they're a game-changer. These concise expressions allow you to create lists with a single line of code, unlocking the potential for streamlined and readable solutions.
Why it matters: In data processing, list comprehensions can significantly reduce code complexity and enhance code maintainability.
# Traditional approach
squared_numbers = []
for num in range(1, 6):
squared_numbers.append(num ** 2)
# Using list comprehension
squared_numbers = [num ** 2 for num in range(1, 6)]
The Anatomy of List Comprehensions: Break it Down
List comprehensions consist of three fundamental components: the expression, the iterator, and the optional condition. Understanding this structure is key to mastering this Pythonic technique.
How it works: We'll dissect the anatomy of list comprehensions to show you how each component contributes to creating efficient code.
# Expression: num ** 2
# Iterator: num
# Condition (optional): if num % 2 == 0
squared_evens = [num ** 2 for num in range(1, 6) if num % 2 == 0]
List Comprehensions in Action: Real-World Use Cases
List comprehensions find applications in various domains. We'll explore five distinct scenarios where list comprehensions shine, accompanied by practical examples:
1. Filtering Data: Efficiently extract data that meets specific criteria.
# Find even numbers in a list
even_numbers = [num for num in numbers if num % 2 == 0]
2. Transforming Data: Easily modify data elements to meet your needs.
# Convert names to uppercase
uppercase_names = [name.upper() for name in names]
3. Nested Lists: Navigate and manipulate nested lists with ease.
# Flatten a list of lists
flattened_list = [item for sublist in nested_list for item in sublist]
4. Dictionary Comprehensions: Generate dictionaries using the same concise syntax.
# Create a dictionary of squares
squares_dict = {num: num ** 2 for num in numbers}
5. Set Comprehensions: Employ list comprehensions to create sets effortlessly.
# Remove duplicate elements from a list
unique_elements = {element for element in elements}
Advantages of List Comprehensions: Why They Matter
List comprehensions offer several advantages, including improved code readability, reduced code length, and enhanced performance. We'll showcase these benefits through practical examples and comparisons.
How it helps: Learn why list comprehensions matter and how they can make your code more efficient.
# Traditional approach
squared_numbers = []
for num in range(1, 6):
squared_numbers.append(num ** 2)
# Using list comprehension
squared_numbers = [num ** 2 for num in range(1, 6)]
Beyond Basics: Advanced List Comprehension Techniques
Explore advanced techniques that take list comprehensions to the next level:
1. Multiple Iterators: Combine multiple iterators to solve complex problems.
# Create pairs of numbers from two lists
pairs = [(x, y) for x in list1 for y in list2]
2. Conditional Expressions: Utilize conditional expressions for more precise filtering.
# Replace negative values with zero
filtered_values = [value if value >= 0 else 0 for value in data]
3. Nested List Comprehensions: Nest comprehensions for multi-dimensional data manipulation.
# Transpose a matrix
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
transposed = [[row[i] for row in matrix] for i in range(len(matrix[0]))]
Conclusion: Elevate Your Python Coding Game
As we conclude our exploration of Python list comprehensions, remember that they are not just a feature; they're a coding superpower. Embrace list comprehensions, and you'll find yourself writing cleaner, more efficient, and more elegant Python code.
Unlock the power of list comprehensions in your Python journey, and join us for the next issue, where we'll delve into another exciting aspect of Pythonic coding.
Stay Pythonic,
Geek Verma
P.S. Don't miss our next issue, where we'll dive into the world of advanced Pythonic coding techniques!
Reply