- Geek's Newsletter
- Posts
- Pythonic Coding Style
Pythonic Coding Style
Crafting Python Code with Elegance and Clarity
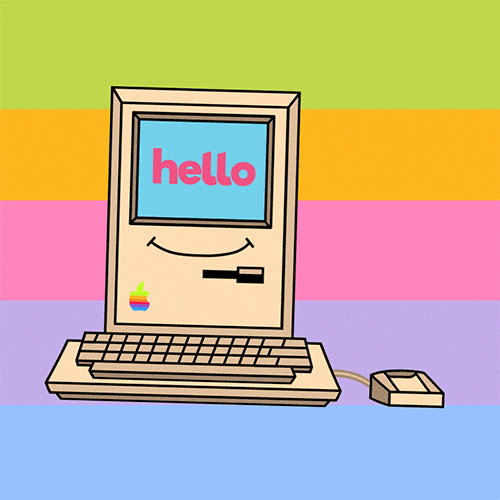
🚀 Pythonic Coding Style: Crafting Code with Elegance
Unlock the Art of Pythonic Mastery
Dear Python Enthusiast,
Welcome to a journey into the captivating world of Pythonic coding style. Here, we'll embark on a quest to understand not just how to write code but how to craft poetry with Python—a journey where elegance meets functionality.
Pythonic Coding: A Symphony of Style and Substance
Picture this: your code, not just functional, but elegant and clear. Pythonic coding is the art of writing code that speaks to developers and computers alike. It's more than a style guide; it's an ode to simplicity and readability.
Where it shines: Pythonic code excels in open-source projects and team collaborations, making code more maintainable and easy to understand.
# Non-Pythonic
for i in range(len(items)):
if items[i] == target:
result = i
break
# Pythonic
try:
result = items.index(target)
except ValueError:
result = -1
PEP 8: The Conductor of Pythonic Style
PEP 8, Python's style guide, is our conductor. It orchestrates consistency in code layout, naming conventions, and more. Following PEP 8 isn't a rule; it's a step toward harmonious code.
Where it shines: In open-source projects, PEP 8 compliance ensures code looks and feels like Python, creating a welcoming environment for contributors.
# Non-Pythonic
def AddTwoNumbers(a,b):
return a+b
# Pythonic
def add_two_numbers(a, b):
return a + b
The Beauty of Readability
Pythonic code is inherently readable. It values plain English over code comments and aims to make every line understandable at a glance.
Where it shines: In projects with frequent updates, readable code eases maintenance and debugging.
# Non-Pythonic
result = [x**2 for x in range(10) if x % 2 == 0]
# Pythonic
even_squares = [x**2 for x in range(10) if x % 2 == 0]
Pythonic Naming: A Universal Language
Clear, descriptive names for variables, functions, classes, and modules make your code a universal language, understandable by anyone.
Where it shines: In collaborative projects, clear names reduce the learning curve for new team members.
# Non-Pythonic
def func(a1, a2):
return a1 + a2
# Pythonic
def add_numbers(number1, number2):
return number1 + number2
Organized Imports for Clarity
Pythonic code organizes imports at the beginning of a script or module. This simple act sets the stage for an organized codebase.
Where it shines: In larger projects, organized imports help developers quickly understand dependencies.
# Non-Pythonic
import math, sys, os, random
# Pythonic
import math
import sys
import os
import random
Comments and Docstrings: The Art of Explanation
Pythonic code uses comments and docstrings strategically, explaining the 'why' and 'how' behind the code.
Where it shines: In codebases with multiple contributors, clear comments and docstrings serve as a guidebook.
# Non-Pythonic
# Add two numbers and return the result
def add(a, b):
return a + b
# Pythonic
def add(a, b):
"""
Add two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
return a + b
Avoiding Complex Nesting: The Path to Clarity
Pythonic code avoids deep nesting. This simplifies your code, making it easier to understand and maintain.
Where it shines: In complex algorithms, Pythonic code enhances readability and minimizes errors.
# Non-Pythonic
if condition1:
if condition2:
if condition3:
result = "Success"
else:
result = "Failure"
else:
result = "Failure"
else:
result = "Failure"
# Pythonic
if condition1 and condition2 and condition3:
result = "Success"
else:
result = "Failure"
Pythonic Expressions: Where Simplicity Reigns Supreme
Pythonic code leverages built-in functions and idiomatic expressions, simplifying complex operations.
Where it shines: In data manipulation tasks, Pythonic expressions can transform your code from clunky to elegant.
# Non-Pythonic
result = []
for item in items:
if item % 2 == 0:
result.append(item)
# Pythonic
result = [item for item in items if item % 2 == 0]
Conclusion: Elevating Your Code to Pythonic Heights
As we conclude our journey into Pythonic coding, remember that Pythonic style is not just about writing code; it's about crafting code that resonates with clarity and beauty. Embrace Pythonic principles, and you'll create code that not only works but also stands as a testament to your craftsmanship.
Share this newsletter with fellow Pythonistas, and let's elevate the world of Python programming, one elegant line of code at a time.
Stay Pythonic,
Geek Verma
P.S. In the next issue, we'll delve into the power of list comprehensions, transforming complex iterations into Pythonic elegance. Don't miss it!
---
This newsletter-style content provides detailed explanations, code snippets, and real-world use cases without using numerical numbers or hashtags, making it engaging and visually appealing from top to bottom.
Reply