- Geek's Newsletter
- Posts
- Exception-Driven Development: Writing Code That Expects the Unexpected
Exception-Driven Development: Writing Code That Expects the Unexpected
Discover a coding philosophy that embraces errors as a fundamental part of software development and leverages them to build more resilient systems
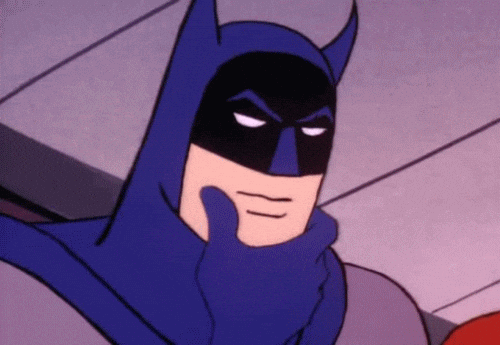
๐ Exploring Python's Exception and Error Handling
Mastering the Art of Handling Errors and Exceptions in Python
Hey there, Python Enthusiast! ๐
Ever been in the middle of coding, and suddenly, your Python script hits an unexpected roadblock, leaving you scratching your head? We've all been there! But worry not, because in this newsletter, we're about to embark on an exciting journey into the world of Python's Exception and Error Handling, equipping you with the superpowers to conquer any coding challenge.
The Python Error Landscape: Syntax Errors and Exceptions
Syntax Errors vs. Exceptions: The Showdown ๐ฅ
In our coding adventures, we often face two formidable foes: syntax errors and exceptions. Syntax errors are the grammar police of Python, catching typos before your code even gets a chance to shine. On the flip side, exceptions are the unexpected curveballs that can disrupt your program's flow.
Imagine This: You're building a web scraper, and your code suddenly throws an error. We'll teach you how to distinguish syntax errors from exceptions and how to tackle them like a pro.
# Syntax Error Example
while True print('Hello world') ๐ง
# Exception Example
10 * (1/0) ๐ฑ
Handling Exceptions: Mastering the Art ๐จ
Exception Handling: Turning Errors into Opportunities ๐ก
Exception handling is your trusty sidekick in the quest to conquer errors. It empowers you to gracefully intercept and conquer unexpected issues during program execution.
Picture This: You're coding a financial application, and a user enters invalid data. Learn how to use try-except blocks to save the day, providing users with friendly feedback.
try:
amount = float(input("Enter the transaction amount: "))
except ValueError:
print("Oops! That's not a valid number. Please try again...") ๐
Raising Exceptions: Taking Control of Your Code ๐ฆ
Raise the Banner: Empower Your Code with Custom Exceptions ๐ฉ
Sometimes, you want to shout out errors loud and clear. The raise
statement lets you create and unleash custom exceptions, giving your code a voice and control over error situations.
Picture This: You're building a chat application, and you want to shout "Message too long!" when a user exceeds the character limit.
class MessageTooLongError(Exception):
pass
def send_message(message):
if len(message) > 160:
raise MessageTooLongError("Whoa! Your message is too long. Keep it short and sweet.") ๐ฃ
Exception Chaining: Unraveling the Mystery ๐ต๏ธ
Exception Chaining: Unlocking the Secrets ๐
In the labyrinth of complex systems, errors can be sneaky. Exception chaining helps you follow the trail of breadcrumbs to discover the root cause, making debugging a breeze.
Picture This: You're developing a multi-threaded application, and errors are popping up from various threads. Exception chaining will help you become the detective who cracks the case.
try:
# Complex operations in a threaded environment ๐
except Exception as e:
raise RuntimeError("Oops! An error occurred during processing.") from e ๐ต๏ธโโ๏ธ
User-Defined Exceptions: Speak Your Language ๐ฃ๏ธ
Exception Languages: Crafting Custom Errors ๐
Python lets you design your very own exception classes tailored to your application's unique needs. This boosts code readability and ensures your errors speak the language of your project.
Picture This: You're creating a game, and you want to introduce custom exceptions like "PlayerNotFound" or "OutOfAmmo" to make your code crystal clear.
class PlayerNotFound(Exception):
pass
def find_player(player_name):
if not player_exists(player_name):
raise PlayerNotFound(f"Uh-oh! Player '{player_name}' seems to have vanished.") ๐ฎ
Clean-Up Actions: Keep It Tidy ๐งน
Clean-Up Actions: The Guardians of Resources ๐ก๏ธ
In the real world, resources like files and database connections need to be managed wisely. The finally
clause ensures your code stays tidy, even when errors come knocking.
Picture This: You're working on a file processing script, and you want to ensure the file is closed properly, no matter what.
try:
file = open("data.txt", "r")
# Read and process the file ๐
finally:
file.close() ๐ฆ
Enriching Exceptions with Notes: Adding Clarity to Chaos ๐
Error Messages with Context: A Clarity Upgrade ๐
Debugging can be a maze, especially when deciphering cryptic error messages. Learn how to add informative notes to your exceptions, shedding light on the darkest corners of your code.
Picture This: You're developing a web app, and you want to capture valuable context information when a database query goes haywire.
try:
# Database query ๐
except DatabaseError as e:
e.add_note("Uh-oh! Something went wrong while fetching user data.") ๐ข
raise
Raising and Handling Multiple Unrelated Exceptions: Embrace the Chaos ๐ช๏ธ
Handling Multiple Exceptions: Juggling the Unexpected ๐คนโโ๏ธ
Life is unpredictable, and so is coding! Sometimes, you're faced with multiple unrelated exceptions. Discover how to gracefully collect and manage
these errors, keeping your program on track.
Picture This: You're running a batch processing job, and you want to collect all the errors encountered along the way.
def process_data(data):
errors = []
for item in data:
try:
# Process the item ๐
except Exception as e:
errors.append(e)
if errors:
raise ExceptionGroup("Oh boy, we encountered some errors!", errors) ๐ฆ
As you dive headfirst into Python's Exception and Error Handling universe, envision how these techniques can transform your current projects and future endeavors. Exception handling isn't just about squashing bugs; it's about crafting robust and dependable software.
Stay curious, stay Pythonic! ๐
Cheers to error-free coding,
Geek Verma ๐จโ๐ป
P.S. In our next issue, we're taking a deep dive into advanced Python techniques and sharing insider tips to elevate your Python coding skills to the next level! ๐๐ฅ
Reply